Multi-touch gestures are very useful in many common scenarios, such as zooming, panning. For game development, handling multi-touch is essential. This post will give a quick guide for handling multi-touch points.
Project structure
We create a new project name MultiTouch
with Java and add a new class named GameScene
as a subclass of android.view.View
.
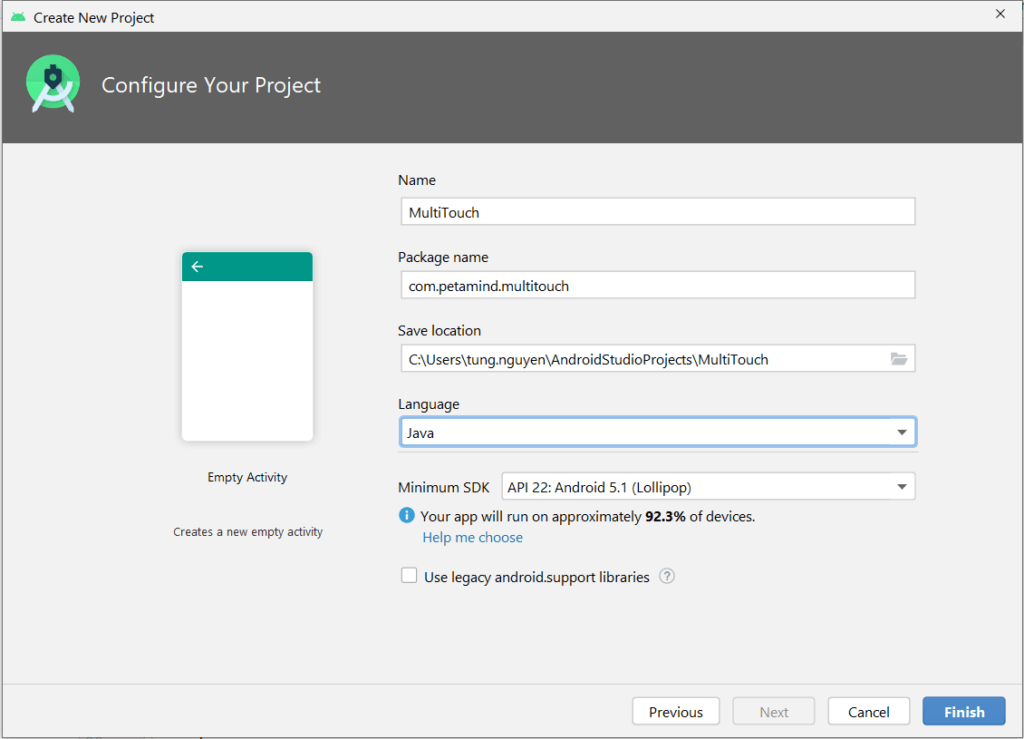
The basic GameScene
class is as follows.
public class GameScene extends View {
public GameScene(Context context) {
super(context);
}
}
And to use GameScene
as the main view of our demo. We set it in MainActivity as follows.
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(new GameScene(this));
}
}
The task
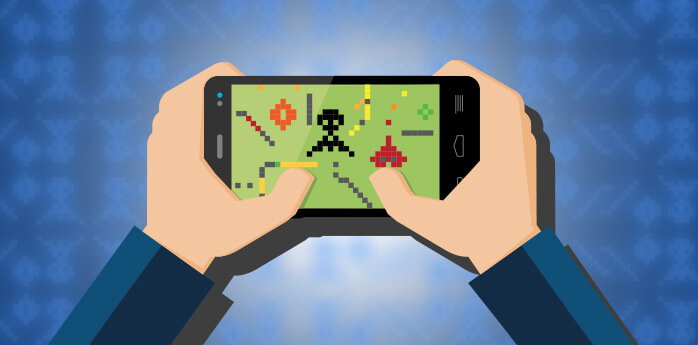
We will now modify the `GameScene
so that it will display the location info of touched points. You can then modify your own logic to achieve your own goal.
We will need:
- Array to store touched points: SparseArray<PointF>
- A paint to draw text on the screen
We initialize these in the constructor
public class GameScene extends View {
private SparseArray<PointF> mActivePointers;
private Paint paint;
public GameScene(Context context) {
super(context);
mActivePointers = new SparseArray<PointF>();
paint = new Paint();
paint.setTextSize(50);
}
}
Next, we handle the touch event by adding all touched points to the array and remove it when a finger leaves the screen
@Override
public boolean onTouchEvent(MotionEvent event) {
// get pointer index from the event object
int pointerIndex = event.getActionIndex();
// get pointer ID
int pointerId = event.getPointerId(pointerIndex);
// get masked (not specific to a pointer) action
int maskedAction = event.getActionMasked();
switch (maskedAction) {
case MotionEvent.ACTION_DOWN:
case MotionEvent.ACTION_POINTER_DOWN: {
PointF f = new PointF();
f.x = event.getX(pointerIndex);
f.y = event.getY(pointerIndex);
mActivePointers.put(pointerId, f);
break;
}
case MotionEvent.ACTION_MOVE: { // a pointer was moved
for (int size = event.getPointerCount(), i = 0; i < size; i++) {
PointF point = mActivePointers.get(event.getPointerId(i));
if (point != null) {
point.x = event.getX(i);
point.y = event.getY(i);
}
}
break;
}
case MotionEvent.ACTION_UP:
case MotionEvent.ACTION_POINTER_UP:
case MotionEvent.ACTION_CANCEL: {
mActivePointers.remove(pointerId);
break;
}
}
postInvalidate();
//redraw the gameview
return true;
}
Finally, we draw text based on the touch points.
@Override
protected void onDraw(Canvas canvas) {
super.onDraw(canvas);
canvas.drawColor(Color.WHITE);
// draw all pointers
for (int size = mActivePointers.size(), i = 0; i < size; i++) {
PointF point = mActivePointers.valueAt(i);
canvas.drawText(point.toString(), point.x , point.y - 50, paint);
}
}
The multi-touch result
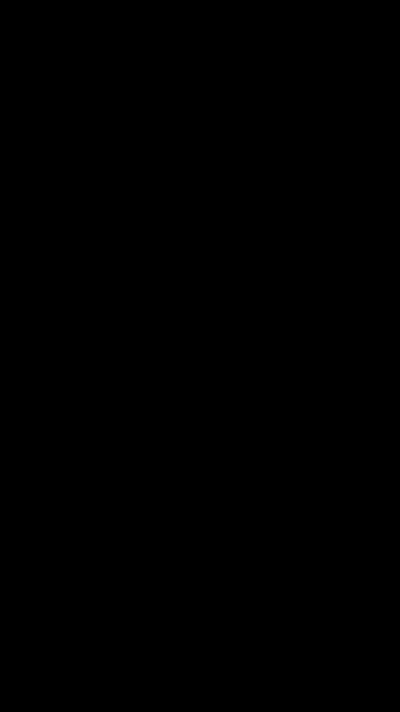
Why do we have to use multi-touch when itβs about game? Can you explain?
It is obvious but hard to explain π