A simple game does not always come with simple implementation w.r.t character animations. This articles will introduce a practical design pattern in game development to deal with this.
How many animations in this game scene
Let have a look at the famous game scene of flappy bird and try to identify how many animations.
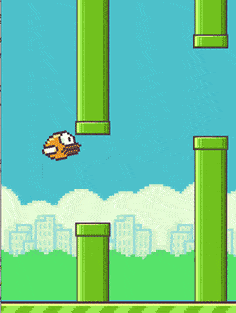
Visually, you may say there are two animations: the pipes and the bird. However, programmatically, there are four (4) types of animations to deal with:
- The flapping animation (fig 2a)
- The up and down movement (fig 2b)
- The pipes movement (fig 2c)
- The ground movement (fig 2d)
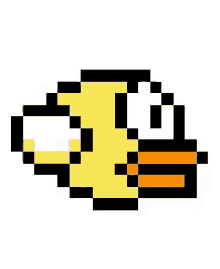
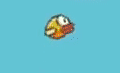
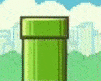

Is that too many animations in a game scene?!! How can you handle them effectively? Don’t panic! If we can identify movements like that then it already helps the coding parts become much clearer.
Animation principle
By definition, the animation is a method in which pictures are manipulated to appear as moving images. The human visual system can process 10 to 12 images per second and perceive them individually, while higher rates are perceived as motion [2].
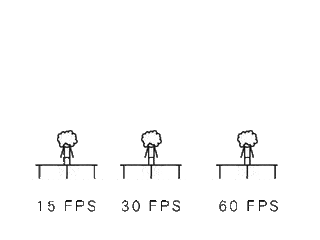
The above figure shows the difference between frame rates. The 3 jumping boys’ moves do not look the same but you may find that they have the same start and same stop. It is because they are sampled from the same sequence but with different frame rates. The higher the frame rate, the smoother the motion. The legs’ movements clearly reflect that.

- 1 sec = 1000 millisecond (ms)
- 10 FPS = 10 frames per second. It means we update a frame every 0.1 seconds (100 ms)
- 24 FPS = 24 frames per second. It means we update a frame every 1000/24 = 41.6 ms.
The core frame rate
You may wonder different object may have different frame rate. It is true! For example, you may want the bird to flap every half a second (0.5 sec) but you want the pipes and the ground move every 0.02 sec (2ms) (or 50FPS).
The core frame rate is the rate that makes sure all objects’ updates are visible. Namely, it should be at least equal the highest frame rate of an object in a game scene.
As suggested, we should use a frame rate from 24fps to 60fps for covering most common types of animation, e.g. walk, run, candles being blown out, explosion. Recently, most of the HD games set the core frame rate to 60FPS. Why? Let see the short explanation video from Google.
GAME LOOP pattern
The central component of any game, from a programming standpoint, is the game loop. The game loop allows the game to run smoothly regardless of a user’s input or lack thereof. A highly simplified game loop of an action game, in pseudocode, might look something like this :
while( user doesn't exit )
check for user input
run AI
move enemies
resolve collisions
draw graphics
play sounds
end while
#src: WIKIPEDIA
It depends on the game genre to decide how to implement a game loop. The most basic methods you should include in a game loop are the draw (render) and update (e.g. move enemies, move a character, check collisions, etc.).
The duration to update a game scene frame is, of course, the core frame rate. And there is a couple of ways to implement this. One example is to ask the current thread to sleep for a while and then update. Sample code:
//MS_PER_FRAME is the duration (in millisecond) to display a frame.
//MS_PER_FRAME = 1000/FRAME_RATE
while (true)
{
double start = getCurrentTime();
processInput();
update();
render();
sleep(start + MS_PER_FRAME - getCurrentTime());
}
There are some drawbacks of this method. For example, if it takes longer than MS_PER_FRAME to update
and render
the frame, your sleep time goes negative.
It is suggested to implement smarter update like this: a certain amount of real-time has elapsed since the last turn of the game loop. This is how much game time we need to simulate for the game’s “now” to catch up with the player’s. We do that using a series of fixed time steps. The code looks a bit like:
double previous = getCurrentTime();
double lag = 0.0;
while (true)
{
double current = getCurrentTime();
double elapsed = current - previous;
previous = current;
lag += elapsed;
processInput();
while (lag >= MS_PER_UPDATE)
{
update();
lag -= MS_PER_UPDATE;
}
render();
}
There are a few pieces here. At the beginning of each frame, we update lag
based on how much real-time passed. This measures how far the game’s clock is behind compared to the real world. We then have an inner loop to update the game, one fixed step at a time, until it’s caught up. Once we’re caught up, we render and start over again. The visualization of the process is like this:

To Sum up
It is good to understand the foundation of animation before using a game engine. When the game become larger, it is not easy to manage all objects’ animations. We handle them effectively with good programming pattern such as game loop and break down complex animations into several smaller parts.
The next article will detail the implementation of a game scene with the mentioned technique.
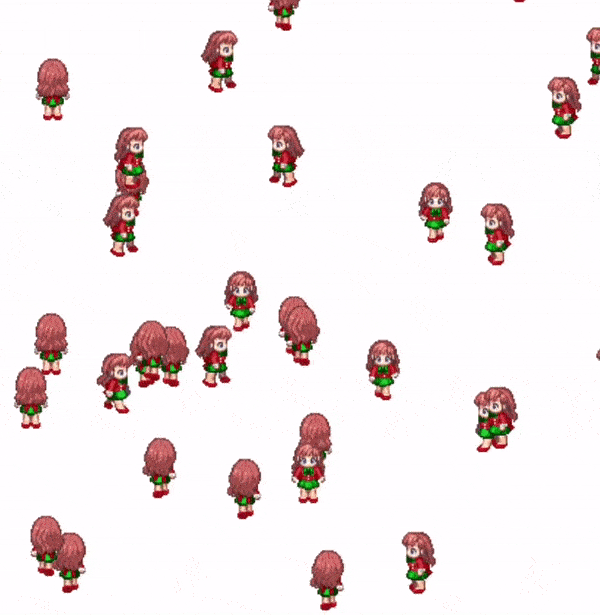
References:
[1]. Game loop, https://gameprogrammingpatterns.com/game-loop.html.
[2]. Game programming, https://en.wikipedia.org/wiki/Game_programming.
[3]. Smooth snake, https://petamind.com/smooth-nature-snake-game-redev-with-kotlin-part-3/.
Very interesting! Looking forward to reading your next article! Learning about games is fun! 😄
[…] class renders points on 2D surface view. We use a simple and lazy technique described in this article. By default, the constructor takes 100 points on the sphere. We declare radius as 1/3 of the screen […]
[…] part 1, we covered the principle of game frame rate and a useful game programming pattern for performance. […]