Printing is an important part when programming. Printing the US flag to the system console was a small part of “the shape game” for kids, in which the players will select the correct name for a given shape.
Basic Requirements:
You are required to print the American flag to the console using just “*” and “=”. The number of stars varies depending the line (row). A sample output:
* * * * * * ==================================
* * * * * ==================================
* * * * * * ==================================
* * * * * ==================================
* * * * * * ==================================
* * * * * ==================================
* * * * * * ==================================
* * * * * ==================================
* * * * * * ==================================
==============================================
==============================================
==============================================
==============================================
==============================================
==============================================
Fun facts of students’ solutions
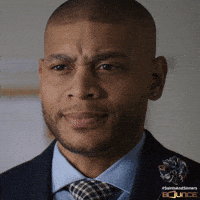
Most of the students provided solutions which the flag is hardcoded. It means they fixed (data or parameters) in a program in such a way that they cannot be altered without modifying the program. A typical solution (in JAVA) looks like this:
public class USAFlag {
public static void main(String[] args)
{
System.out.println("* * * * * * ==================================");
System.out.println(" * * * * * ==================================");
System.out.println("* * * * * * ==================================");
System.out.println(" * * * * * ==================================");
System.out.println("* * * * * * ==================================");
System.out.println(" * * * * * ==================================");
System.out.println("* * * * * * ==================================");
System.out.println(" * * * * * ==================================");
System.out.println("* * * * * * ==================================");
System.out.println("==============================================");
System.out.println("==============================================");
System.out.println("==============================================");
System.out.println("==============================================");
System.out.println("==============================================");
System.out.println("==============================================");
}
}
Or partly hard-coded 😀 . Printing the first few rows are tricky as they are mixed “*” and “=”. Therefore, they decided to store the two lines (of the flag) in a variable.
public static void main(String[] args) {
String p1 = "* * * * * * ==================================\n * * * * * ==================================";
String p2 = "==============================================";
for (int i = 0; i < 4; i++) {
System.out.println(p1);
}
System.out.println("* * * * * * ==================================");
for (int i = 0; i < 6; i++) {
System.out.println(p2);
}
}
The advanced requirements:
The previous code works perfectly but it is not flexible. Now let make it a bit more challenging by some new requirements:
- No more hard-coded
- Make the flag scalable with the width and height (as integers)
- Make the code as short as possible.
Give yourself 5 mins before going to the following solution.
Print flag Using Java
We use loops to print line by line. First, we print star patterns, then we print “=”, finally we print new line.
The default width and height are 46 and 15 respectively. The star pattern is 6* on even index lines (if the first line has index 0) and 5* on odd index lines. Between 2 stars, we have a space (” “). Therefore, all the stars are bounded in 9×12 rectangle. We print a star only when the sum of the row and column indices are ODD.
An easy to understand version:
public static void main(String[] args) {
for (int i = 0; i < 15; i++) {
for (int j = 0; j < 46; j++) {
if(i < 9 && j < 12){
if((i+j)%2 == 0 && j != 11){
System.out.print("*");}
else {
System.out.print(" ");}
} else {
System.out.print("=");
}
}//End for
System.out.println();
}//End for
}
A crazy version with only ONE print line.
For scalability, we create a new function, printUSAFlag, that takes 2 input params height and width. The indices i and j are controlled with ratio to the original width and height. Now we try to shorten the code with the same logic by replacing if-statements with the ternary operator. Syntax:
condition ? expr1 : expr2
#condition—An expression that evaluates to true or false
#expr1, expr2—Expressions with values of any type
If condition is true, the value of expr1 is returned; if condition is false, the value of expr2 is returned.
public static void printUSAFlag( int height, int width) {
for (int i = 0; i < height; i++) {
for (int j = 0; j < width; j++) {
System.out.print(((i< 9*height/15 && j < 12*width/46 )?
(((i+j)%2==0 && j != 12*width/46 - 1)?"*":" "):"=" )+(j==width-1?"\n":""));
}
}
}
printUSAFlag(5,16)
#output:
* * *===========
* * ===========
* * *===========
================
================
Print with Python
With the same logic, we can implement with Python.
def printUSAFlag(height, width):
s = ""
for i in range(height):
for j in range(width):
s+=(('*' if ((i+j)%2==0 and j!= 12*width/46 -1) else " ") if (i < 9*height/15 and j <12*width/46) else "=") + ('\n' if j==width-1 else '')
print(s)
Want to play with python a little more with python generator?
def printUSAFlag(height, width):
print(''.join([(('*' if ((i+j)%2==0 and j!= 12*width/46 -1) else " ")
if (i < 9*height/15 and j <12*width/46) else "=") +
('\n' if j==width-1 else '') for i in range(height) for j in range(width)]))
Print Flag with Kotlin
If you are new to Kotlin, you can read this quick tutorial.
fun printUSAFlag(height: Int, width: Int) {
for (i in 0 until height) {
for (j in 0 until width) {
print(
(if (i < 9 * height / 15 && j < 12 * width / 46)
if ((i + j) % 2 == 0 && j != 12 * width / 46 - 1) "*" else " "
else
"=") + if (j == width - 1) "\n" else ""
)
}
}
}
Now we can test with any width and height.
fun main() {
printUSAFlag(6, 18)
}
* * ==============
* ==============
* * ==============
==================
==================
==================
I love all your blogposts! Not just the content but also the way you deliver it!
Thanks for your words. It is really encouraging. 😀